Introduction
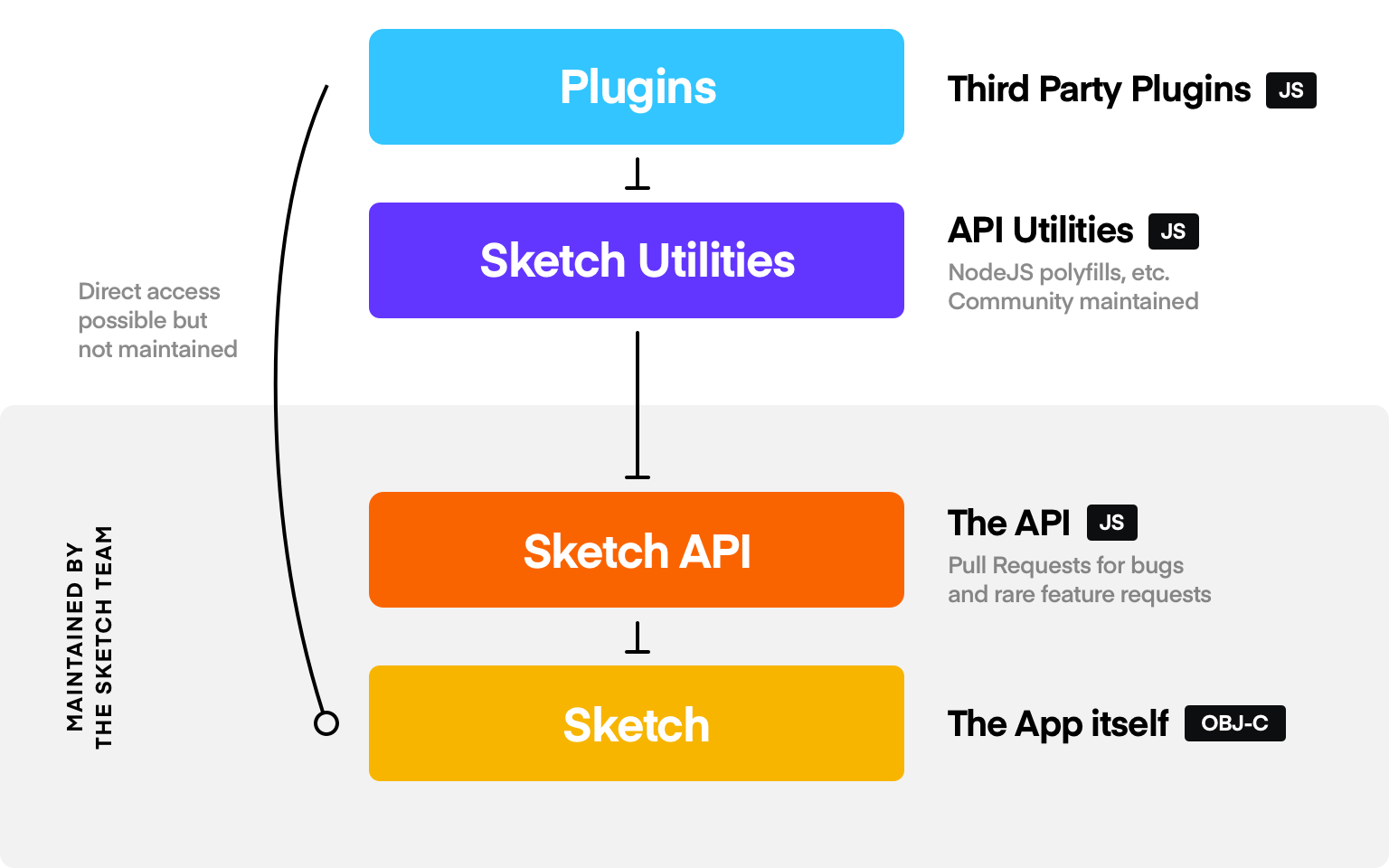
This is a prototype Javascript API for Sketch. It’s still a work in progress, but the intention is to make something which is:
- native Javascript
- an easily understandable subset of the full internals of Sketch
- fully supported by Sketch between releases (ie we try not to change it, unlike our internal API which we can and do change whenever we need to)
- still allows you to drop down to our internal API when absolutely necessary.
The idea is to keep this API as lean-and-mean as possible. The main reason for that is that if we (Sketch) are committing ourselves to making sure that we don’t break it, then it’s essential that doing so doesn’t become an unmanageable task.
Again, this is very much a work in progress, so comments and suggestions for this API are welcome - send them to developer@sketch.com, or open an issue to have a public discussion.
Installation
var sketch = require('sketch/dom')
var async = require('sketch/async')
var DataSupplier = require('sketch/data-supplier')
var UI = require('sketch/ui')
var Settings = require('sketch/settings')
// a more convenient require which exposes everything (might be a bit slower)
var sketch = require('sketch')
The API comes bundled inside Sketch, so no installation is required. You access it by calling the global require
function.
Sketch Components
The approach taken by the API is to wrap the native Sketch model objects inside javascript objects. These are thin wrappers, and contain no state - they just exist as a way to provide a cleaner and more stable coding interface to the underlying model.
Each Component follows the same API:
Component.fromNative(sketchObject)
returns a wrapped object from a native Sketch model objectComponent.toJSON()
return a JSON object that represent the componentnew Component(properties)
creates a new native Sketch model object and returns a wrapped objectcomponentInstance.sketchObject
returns the native Sketch model object.componentInstance.isImmutable()
returnstrue
if the component is wrapping an immutable version of a native Sketch model object. If that is the case, you won’t be able to mutate the object (setting any property will be a no-op).componentInstance.type
returns a string that represent the type of the component. If it’sundefined
, it means that we couldn’t match the native object and that we returned a really lightweight wrapper.
Document
var Document = require('sketch/dom').Document
A Sketch document.
Properties | |
---|---|
idstring | The unique ID of the document. |
pagesPage[] | The pages of the document. |
selectedPagePage | The selected page of the Document. |
selectedLayersSelection | The Selection of the layers that the user has selected in the currently selected page. |
pathstring | The path to the document (or the appcast URL in case of a Document from a remote Library). |
sharedLayerStylesSharedStyle[] | The list of all shared layer styles defined in the document. |
sharedTextStylesSharedStyle[] | The list of all shared text styles defined in the document. |
colorsColorAsset[] | A list of color assets defined in the document. Mutating the returned array will update the document colors. |
swatchesSwatch[] | A list of swatches defined in the document. Mutating the returned array will update the document swatches. |
gradientsGradientAsset[] | A list of gradient assets defined in the document. Mutating the returned array will update the document gradients. |
colorSpaceColorSpace | The color space of the document. |
Access the selected Document
var document = require('sketch/dom').getSelectedDocument()
// also exposed on Document
var document = Document.getSelectedDocument()
Returns
The selected Document or undefined
if no document is open.
Access all the open Documents
var documents = require('sketch/dom').getDocuments()
// also exposed on Document
var documents = Document.getDocuments()
Returns
An array of Documents.
Create a new Document
var document = new Document()
Open a Document
// ask the user to select a document
Document.open((err, document) => {
if (err) {
// oh no, we failed to open the document
}
// if the user cancel the opening, `document` will be `undefined`
})
// open a sketch document at the specified path
Document.open('path/to/the/document.sketch', (err, document) => {
if (err) {
// oh no, we failed to open the document
}
})
A method to open an existing sketch document or ask the user to open one. The method is asynchronous so if you want to do something after the document is opening it, make sure that you pass a callback and continue your script there.
Parameters | |
---|---|
pathstring | The path to the document to open. If undefined , the user will be asked to select one. |
callbackfunction | A function called after the document is opened. It is called with an Error if opening the Document was unsuccessful and a Document (or undefined ). |
Find a Layer by Id
var layer = document.getLayerWithID(layerId)
if (layer) {
// do something
}
A method to help find the first layer in this document which has the given id.
Parameters | |
---|---|
layerIdstring - required | The ID of the layer to find |
Returns
Return a Layer object or undefined
if it’s not found.
Find Layers by name
var layers = document.getLayersNamed(name)
if (layers.length) {
// do something
}
A method to help find the layers in this document which have the given name.
Parameters | |
---|---|
namestring - required | The name of the layers to find |
Returns
Return an array of Layer.
Find a Shared Layer Style
var sharedStyle = document.getSharedLayerStyleWithID(sharedStyleId)
A method to help find a shared style in the document.
Parameters | |
---|---|
sharedStyleIdstring - required | The ID of the shared style to find |
Returns
Return a SharedStyle object or undefined
if it’s not found.
Find a Shared Text Style
var sharedStyle = document.getSharedTextStyleWithID(sharedStyleId)
A method to help find a shared style in the document.
Parameters | |
---|---|
sharedStyleIdstring - required | The ID of the shared style to find |
Returns
Return a SharedStyle object or undefined
if it’s not found.
Get all the Symbol Sources
var symbols = document.getSymbols()
A method to get all Symbol Sources defined in the document.
Returns
Return an array of the SymbolMaster objects defined in the document.
Find a Symbol Source
var source = document.getSymbolMasterWithID(symbolInstance.symbolId)
A method to help find a Symbol Source in the document.
Parameters | |
---|---|
symbolIdstring - required | The Symbol ID of the Symbol Source to find |
Returns
Return a SymbolMaster object or undefined
if it’s not found.
Center on Layer
document.centerOnLayer(layer)
A method to help center the view of the document window on a given layer.
Parameters | |
---|---|
layerLayer - required | The layer to center the view onto |
Save the Document
document.save()
document.save('path/to/the/document.sketch')
document.save('path/to/the/document.sketch', (err) => {
if (err) {
// saving the document failed :(
}
})
A method to save a document to a specific path or ask the user to choose where to save it. The method is asynchronous so if you want to do something after the document is saved, make sure that you pass a callback and continue your script there.
Parameters | |
---|---|
pathstring | The path where the document will be saved. If undefined , the user will be asked to select one. |
optionsobject | The options for the save operation (only used when specifying a path). |
options.saveModeSaveMode | The way to save the document. |
callbackfunction | A function called after the document is saved. It is called with an Error if saving the Document was unsuccessful. |
Close the Document
document.close()
A method to close a document.
Change the color space
// By default the method assigns a new color space
document.changeColorSpace(ColorSpace.sRGB)
console.log(document.colorSpace === ColorSpace.sRGB) // true
// Pass true as an optional second argument
// to convert instead of assign
document.changeColorSpace(ColorSpace.P3, true)
console.log(document.colorSpace === ColorSpace.P3) // true
// Alternatively, use the property setter (the behaviour
// here is to always assign the color space)
document.colorSpace = ColorSpace.P3
console.log(document.colorSpace === ColorSpace.P3) // true
// Create a document with a pre-defined color space
const p3Doc = new Document({ colorSpace: ColorSpace.P3 })
A method to change a document’s color space. For an in-depth discussion of this topic and the difference between assigning and converting the color space check the color management documentation.
Document.SaveMode
Document.SaveMode.SaveAs
document.save('path/to/the/document.sketch', {
saveMode: Document.SaveMode.SaveAs,
})
Enumeration of the save mode.
Value | |
---|---|
Save |
Overwrites a document’s file with the document’s contents |
SaveAs |
Writes a document’s contents to a new file and then changes the document’s current location to point to the just-written file |
SaveTo |
Writes a document’s contents to a new file without changing the document’s current location to point to the new file. |
Document.ColorSpace
Document.ColorSpace.Unmanaged
Document.ColorSpace.sRGB
Document.ColorSpace.P3
Enumeration of the available color space settings.
Value | |
---|---|
Unmanaged |
The default setting |
sRGB |
sRGB color profile |
P3 |
Display P3 color profile |
Library
var Library = require('sketch/dom').Library
A Sketch Library.
Properties | |
---|---|
idstring - readonly | The unique ID of the Library. |
namestring - readonly | The name of the Library. |
validboolean - readonly | If Sketch has been able to load the Library. If the library is not valid, the methods will often not be available so always check this field before doing something with a library. |
enabledboolean | If the user has enabled the Library. |
libraryTypeLibraryType - readonly | The type of Library. |
lastModifiedAtDate - readonly | The date at which the library was last updated |
Access all the Libraries
var libraries = require('sketch/dom').getLibraries()
// also exposed on Library
var libraries = Library.getLibraries()
Returns
An array of Libraries.
Get a Library from a path
var library = Library.getLibraryForDocumentAtPath(
'path/to/existing/document.sketch'
)
Get the library for a local Sketch document. If the Document was already added as a Library, it will simply return it. If it is not already a Library, it will be added.
Parameters | |
---|---|
pathstring - required | The path of the Library. |
Returns
The existing Library at the path or a new Library from the document at the path.
Get a remote Library from an RSS feed URL
Library.getRemoteLibraryWithRSS(
'https://url/to/feed/rss.xml',
(err, library) => {
if (err) {
// oh no, failed to load the library
}
}
)
Get the remote library for an RSS feed. If the RSS feed was already added as a Library, it will simply return it. If it is not already a Library, it will be added.
Parameters | |
---|---|
urlstring - required | The URL to the rss feed describing the versions of the library. |
callbackfunction | A function called after the library is added. It is called with an Error if adding the Library was unsuccessful and a Library (or undefined ). |
Remove a Library
library.remove()
A method to remove an existing library.
Get the Library’s Document
var libDocument = library.getDocument()
A library references a Sketch Document and you can access it with this method.
Returns
The Document that the Library references. It can throw an error if the Document cannot be accessed.
Get the Symbols that can be imported
var document = sketch.getSelectedDocument()
var symbolReferences = library.getImportableSymbolReferencesForDocument(
document
)
To import a Symbol from a Library, do not access its Document and look for the Symbol Source directly. Instead, get the Symbol References of the Library and use those to import them.
Those references depends on the document you want to import them into. For example if a document has already imported a symbol, it will reference the local version to keep all the instances in sync.
Returns
An array of Shareable Object that represents the Symbols which you can import from the Library.
Get the Shared Layer Styles that can be imported
var document = sketch.getSelectedDocument()
var stylesReferences = library.getImportableLayerStyleReferencesForDocument(
document
)
To import a shared style from a Library, do not access its Document and look for the SharedStyle directly. Instead, get the Shared Layer Style References of the Library and use those to import them.
Those references depends on the document you want to import them into. For example if a document has already imported a shared style, it will reference the local version to keep all the instances in sync.
Returns
An array of Shareable Object that represents the Shared Layer Styles which you can import from the Library.
Get the Shared Text Styles that can be imported
var document = sketch.getSelectedDocument()
var stylesReferences = library.getImportableTextStyleReferencesForDocument(
document
)
To import a shared style from a Library, do not access its Document and look for the SharedStyle directly. Instead, get the Shared Text Style References of the Library and use those to import them.
Those references depends on the document you want to import them into. For example if a document has already imported a shared style, it will reference the local version to keep all the instances in sync.
Returns
An array of Shareable Object that represents the Shared Layer Styles which you can import from the Library.
Get the Shared Swatches that can be imported
var document = sketch.getSelectedDocument()
var stylesReferences = library.getImportableSwatchReferencesForDocument(
document
)
To import a Swatch from a Library, do not access its Document and look for the Swatch directly. Instead, get the Shared Swatch References of the Library and use those to import them.
Those references depends on the document you want to import them into. For example if a document has already imported a shared Swatch, it will reference the local version to keep all the instances in sync.
Returns
An array of Shareable Object that represents the Shared Swatches which you can import from the Library.
Library.LibraryType
Library.LibraryType.LocalUser
Enumeration of the types of Library.
Value |
---|
Internal |
LocalUser |
RemoteUser |
RemoteTeam |
RemoteThirdParty |
Importable Object
var symbolReferences = library.getImportableSymbolReferencesForDocument(
document
)
An Object that can be imported from a Library. All its properties are read-only.
Properties | |
---|---|
idstring | The unique ID of the Object. |
namestring | The name of the Object. |
objectTypeImportableObjectType | The type of the Object. Will only be Library.ImportableObjectType.Symbol for now. |
libraryLibrary | The Library the Object is part of. |
Import in the Document
var symbol = symbolReference.import()
var style = sharedStyleReference.import()
An Importable Object is linked to a Document so importing it will import it in the said Document.
Returns
If the objectType
of the Object is Symbol
, it will return a Symbol Source which will be linked to the Library (meaning that if the Library is updated, the Symbol Instances created from the Source will be updated as well).
Library.ImportableObjectType
Library.ImportableObjectType.Symbol
Enumeration of the types of Importable Objects.
Value |
---|
Symbol |
LayerStyle |
TextStyle |
Swatch |
Style
var Style = require('sketch/dom').Style
var shape = new Shape({
style: {
borders: [{ color: '#c0ffee' }],
},
})
shape.style.fills = [
{
color: '#c0ffee',
fillType: Style.FillType.Color,
},
]
text.style.textUnderline = 'double dash-dot'
The style of a Layer.
Properties | |
---|---|
opacitynumber | The opacity of a Layer, between 0 (transparent) and 1 (opaque). |
blendingModeBlendingMode | The blend mode used to determine the composite color. |
blurBlur | The blur applied to the Layer. |
fillsFill[] | The fills of a Layer. |
bordersBorder[] | The borders of a Layer. |
borderOptionsBorderOptions | The options that the borders share. |
shadowsShadow[] | The shadows of a Layer. |
innerShadowsShadow[] | The inner shadows of a Layer. |
alignmentAlignment | The horizontal alignment of the text of a Text Layer |
verticalAlignmentVerticalAlignment | The vertical alignment of the text of a Text Layer |
kerningnumber / null |
The kerning between letters of a Text Layer. null means that the kerning will be the one defined by the font. |
lineHeightnumber / null |
The height of a line of text in a Text Layer. null means “automatic”. |
paragraphSpacingnumber | The space between 2 paragraphs of text in a Text Layer. |
textColorstring | A rgba hex-string (#000000ff is opaque black) of the color of the text in a Text Layer. |
fontSizenumber | The size of the font in a Text Layer. |
textTransform‘none’ / ‘uppercase’ / ‘lowercase’ | The transform applied to the text of a Text Layer. |
fontFamilystring | The name of the font family of a Text Layer. 'system' means the font family of the OS ('.SF NS Text' on macOS 10.14). |
fontWeightnumber | The weight of the font of a Text Layer. Goes from 0 to 12, 0 being the thinest and 12 being the boldest. Not every weight are available for every fonts. When setting a font weight that does not exist for the current font family, the closest weight that exists will be set instead. |
fontStyle‘italic’ / undefined | The style of the font of a Text Layer. |
fontVariant‘small-caps’ / undefined | The variant of the font of a Text Layer. |
fontStretch‘compressed’ / ‘condensed’ / ‘narrow’ / ‘expanded’ / ‘poster’ / undefined | The size variant of the font of a Text Layer. |
textUnderlinestring: <line-style> [<line-pattern>] ['by-word'] / undefined where <line-style> can be single / thick / double and <line-pattern> can be dot / dash / dash-dot / dash-dot-dot |
The underline decoration of a Text Layer. |
textStrikethroughstring: <line-style> [<line-pattern>] ['by-word'] / undefined where <line-style> can be single / thick / double and <line-pattern> can be dot / dash / dash-dot / dash-dot-dot |
The strikethrough decoration of a Text Layer. |
fontAxesFontAxes | The axes of the Text Layer font (only available when the font is a variable font). |
Get the default line height
var defaultlineHeight = style.getDefaultLineHeight()
When no line height is specified, style.lineHeight
will be undefined
. You can get the default line height of the font using style.getDefaultLineHeight()
.
Returns
A number if the layer is a Text layer or undefined
.
Check if the Style is in sync with a Shared Style
var isOutOfSync = style.isOutOfSyncWithSharedStyle(sharedStyle)
Returns
Whether the Style has some differences with a Shared Style.
Sync the Style with a Shared Style
style.syncWithSharedStyle(sharedStyle)
The style instance will be updated with the value of the Shared Style.
var sharedStyle = styledLayer.sharedStyle
sharedStyle.style = style
The Shared Style value will be updated with the style.
Style.BlendingMode
Style.BlendingMode.Darken
Enumeration of the blending mode.
Value |
---|
Normal |
Darken |
Multiply |
ColorBurn |
Lighten |
Screen |
ColorDodge |
Overlay |
SoftLight |
HardLight |
Difference |
Exclusion |
Hue |
Saturation |
Color |
Luminosity |
Blur
shape.style.blur = {
center: {
x: 10,
y: 20,
},
type: Style.BlurType.Motion,
motionAngle: 10,
}
An object that represent the blur of the layer.
Properties | |
---|---|
blurTypeBlurType | The type of the blur. |
radiusnumber | The radius of the blur. |
motionAnglenumber | The angle of the blur (only used when the blur type is Motion ). |
centerobject | The center of the blur (only used when the blur type is Zoom . |
center.xnumber | The horizontal coordinate of the center of the blur. |
center.ynumber | The vertical coordinate of the center of the blur. |
enabledboolean | Whether the fill is active or not. |
Style.BlurType
Style.BlurType.Background
Enumeration of the type of a blur.
Value | |
---|---|
Gaussian |
A common blur type that will accurately blur in all directions. |
Motion |
Blur only in one direction, giving the illusion of motion. |
Zoom |
Will blur from one particular point out. |
Background |
This will blur any content that appears behind the layer. |
Fill
shape.style.fills = [
{
color: '#c0ffee',
fillType: Style.FillType.Color,
},
]
An object that represent a Fill. color
, gradient
and pattern
will always be defined regardless of the type of the fill.
Properties | |
---|---|
fillTypeFillType | The type of the fill. |
colorstring | A rgba hex-string (#000000ff is opaque black). |
gradientGradient | The gradient of the fill. |
patternobject | The pattern of the fill. |
pattern.patternTypePatternFillType | How the pattern should fill the layer. |
pattern.imageImageData / null |
The image of tile of the pattern. |
pattern.tileScalenumber | The scale applied to the tile of the pattern. |
enabledboolean | Whether the fill is active or not. |
Style.FillType
Style.FillType.Color
Enumeration of the types of fill.
Value |
---|
Color |
Gradient |
Pattern |
Style.PatternFillType
Style.PatternFillType.Fit
Enumeration of the types of pattern fill.
Value |
---|
Tile |
Fill |
Stretch |
Fit |
Border
shape.style.borders = [
{
color: '#c0ffee',
fillType: Style.FillType.Color,
position: Style.BorderPosition.Inside,
},
]
An object that represent a Border.
Properties | |
---|---|
fillTypeFillType | The type of the fill of the border. |
colorstring | A rgba hex-string (#000000ff is opaque black). |
gradientGradient | The gradient of the fill. |
enabledboolean | Whether the border is active or not. |
positionBorderPosition | The position of the border. |
thicknessnumber | The thickness of the border. |
Style.BorderPosition
Style.BorderPosition.Center
Enumeration of the positions of a border.
Value |
---|
Center |
Inside |
Outside |
BorderOptions
shape.style.borderOptions = {
dashPattern: [20, 5, 20, 5],
}
An object that represent the options that the Borders of the Layer share.
Properties | |
---|---|
startArrowheadArrowhead | The type of the arrow head for the start of the path. |
endArrowheadArrowhead | The type of the arrow head for the start of the path. |
dashPatternnumber[] | The dash pattern of the borders. For example, a dash pattern of 4-2 will draw the stroke for four pixels, put a two pixel gap, draw four more pixels and then so on. A dashed pattern of 5-4-3-2 will draw a stroke of 5 px, a gap of 4 px, then a stroke of 3 px, a gap of 2 px, and then repeat. |
lineEndLineEnd | The type of the border ends (if visible). |
lineJoinLineJoin | The type of the border joins (if any). |
Style.Arrowhead
Style.Arrowhead.OpenArrow
Enumeration of the type of the Arrowhead for line layers.
Value |
---|
None |
OpenArrow |
FilledArrow |
Line |
OpenCircle |
FilledCircle |
OpenSquare |
FilledSquare |
Style.LineEnd
Style.LineEnd.Round
Enumeration of the positions of a border.
Value | |
---|---|
Butt |
This is the default option that’ll draw the border right to the vector point. |
Round |
Creates a rounded, semi-circular end to a path that extends past the vector point. |
Projecting |
Similar to the rounded cap, but with a straight edges. |
Style.LineJoin
Style.LineJoin.Miter
Enumeration of the positions of a border.
Value | |
---|---|
Miter |
This will simply create an angled, or pointy join. The default setting. |
Round |
Creates a rounded corner for the border. The radius is relative to the border thickness. |
Bevel |
This will create a chamfered edge on the border corner. |
Shadow
shape.style.shadows = [
{
color: '#c0ffee',
blur: 3,
},
]
shape.style.innerShadows = [
{
color: '#c0ffee',
blur: 3,
},
]
An object that represent a Shadow.
Properties | |
---|---|
colorstring | A rgba hex-string (#000000ff is opaque black). |
blurnumber | The blur radius of the shadow. |
xnumber | The horizontal offset of the shadow. |
ynumber | The vertical offset of the shadow. |
spreadnumber | The spread of the shadow. |
enabledboolean | Whether the fill is active or not. |
Gradient
shape.style.fills = [
{
fillType: Style.FillType.Gradient,
gradient: {
gradientType: Style.GradientType.Linear,
from: {
x: 0,
y: 0,
},
to: {
x: 50,
y: 50,
},
stops: [
{
position: 0,
color: '#c0ffee',
},
{
position: 0.5,
color: '#0ff1ce',
},
{
position: 1,
color: '#bada55',
},
],
},
},
]
An object that represent a Gradient.
Properties | |
---|---|
gradientTypeGradientType | The type of the Gradient. |
fromPoint | The position of the start of the Gradient |
toPoint | The position of the end of the Gradient. |
aspectRationumber | When the gradient is Radial , the from and to points makes one axis of the ellipse of the gradient while the aspect ratio determine the length of the orthogonal axis (aspectRatio === 1 means that it’s a circle). |
stopsGradientStop[] | The different stops of the Gradient |
Style.GradientType
Style.GradientType.Radial
Enumeration of the type of a Gradient.
Value | |
---|---|
Linear |
Linear gradients tend to be the most common, where two colors will appear at opposite points of an object and will blend, or transition into each other. |
Radial |
A radial gradient will create an effect where the transition between color stops will be in a circular pattern. |
Angular |
This effect allows you to create gradients that sweep around the circumference (measured by the maximum width or height of a layer) in a clockwise direction. |
Gradient Stops
An object that represent a Gradient Stop. Each of colors of a Gradient are represented by a Stop. A Gradient can have as many Stops as you’d like.
Properties | |
---|---|
positionnumber | The position of the Stop. 0 represents the start of the gradient while 1 represent the end. |
colorstring | The color of the Stop |
Font Axes
// Get the axes
const axes = textLayer.style.fontAxes
// If axes is non-null the Text Layer's font
// is a valid variable font recognised by Sketch
if (axes) {
// Mutate the axes object, taking care
// to work within the min and max range
// specified for each axis
axes.Weight.value = axes.Weight.max
axes.Monospace.value = 1
// Apply the new axes to update
// the text layer
textLayer.style.fontAxes = axes
}
The fontAxes
property allows you to adjust the parameters, or “axes”, exposed by variable fonts.
It works by allowing you to get and set an object representing the axes for a Text Layer’s current font. The object will only contain information about the axes supported by the Text Layer’s current font, and these will vary from font to font.
The object is keyed by axis name, and has values with the following structure:
Properties | |
---|---|
idstring | The axis id |
minnumber | The minimum value allowable on the axis |
maxnumber | The maximum value allowable on the axis |
valuenumber | The current axis value |
Shared Style
var SharedStyle = require('sketch/dom').SharedStyle
document.sharedTextStyles.push({
name: 'Header 1',
style: text.style,
})
A shared style (either a layer style or a text style).
Properties | |
---|---|
idstring | The unique ID of the Shared Style. |
styleTypeSharedStyle.StyleType | The type of the Shared Style. |
namestring | The name of the Shared Style. |
styleStyle | The Style value that is shared. |
Note that the
id
of a Shared Style coming from a Library might look like this:FBFF821E-20F3-48C5-AEDC-89F97A8C2344[D1A683E0-5333-4EBE-977C-48F64F934E99]
.If you have a Symbol Instance which has a Layer using a Shared Style from a Library and a Layer in the Document using the same Shared Style from the Library, the style will be imported twice; once for use in the layer and once for use by the foreign Symbol. The reason for this is to do with syncing. If you change the Shared Style in the Library it will cause both the Symbol Instance and the Shared Style to be out-of-date in the document. This will be shown in the component sync sheet, but you can choose only to sync the Shared Style (or the Symbol). Using these “private” Shared Styles means that syncing just the shared style doesn’t implicitly also sync the symbol.
The format of these Symbol private shared style IDs is
SYMBOLID[STYLEID]
Where:STYLEID
is the id of the original Shared Style in the original Library. AndSYMBOLID
is the new symbolId of the foreign Symbol in the destination document.Where we have such as Symbol private style, the same ID will be used both as the local ID and as the remote ID.
Create a new Shared Style from a Style
const newSharedStyle = SharedStyle.fromStyle({
name: 'Header 1',
style: layer.style,
document: document,
})
// you can also push to the shared styles arrays directly
document.sharedTextStyles.push({
name: 'Header 1',
style: text.style,
})
Create a new Shared Style with a specific name in a specific Document.
⚠️You can only insert local shared styles (eg. not linked to a Library).
document.sharedLayerStyles
returns the foreign shared styles (eg. linked to a Library) concatenated with the local shared styles. So if you try to insert a new Shared Style at the beginning (usingunshift
for example), it will end up at the beginning of the local Shared Styles but that might not be the beginning of all the shared styles if there are some foreign.
Get all the Instances
var styles = sharedStyle.getAllInstances()
Returns an array of all instances of the Shared Style in the document, on all pages.
Returns
A Style array.
Get all the Instances’ Layers
var layers = sharedStyle.getAllInstancesLayers()
Returns an array of all layers with a Style which is an instance of the Shared Style in the document, on all pages.
Returns
A Layer array.
Get Library defining the style
var originLibrary = sharedStyle.getLibrary()
If the SharedStyle was imported from a library, the method can be used to:
- know about it
- get the library back
Returns
The Library the Shared Style was defined in, or null
if it is a local shared style.
Sync the local reference with the library version
const success = sharedStyle.syncWithLibrary()
If a Library has some updates, you can synchronize the local Shared Style with the Library’s version and bypass the panel where the user chooses the updates to bring.
Returns
true
if it succeeded.
Unlink the local reference from the library
const success = sharedStyle.unlinkFromLibrary()
You can unlink a Shared Style from the Library it comes from and make it a local Shared Style instead.
Returns
true
if it succeeded.
SharedStyle.StyleType
SharedStyle.StyleType.Text
Enumeration of the type of Shared Style. Unknown
indicates the object is broken and Sketch can’t determine the style type.
Value |
---|
Text |
Layer |
Unknown |
Symbol Override
var overrides = symbolInstance.overrides
A Symbol override. This component is not exposed, it is only returned when accessing the overrides
of a Symbol Instance or Symbol Source. The overrides are not available until after the instance is injected into the document.
Properties | |
---|---|
pathstring | The path to the override. It’s formed by the symbolId of the nested symbols separated by a / . |
propertystring | The property that this override controls. It can be "stringValue" for a text override, "symbolID" for a nested symbol, "layerStyle" for a shared layer style override, "textStyle" for a shared text style override, "flowDestination" for a Hotspot target override or "image" for an image override. |
idstring | The unique ID of the override (${path}_${property} ). |
symbolOverrideboolean | If the override is a nested Symbol override. |
valueString / ImageData | The value of the override which can be change. |
isDefaultboolean | If the override hasn’t been changed and is the default value. |
affectedLayerText / Image / Symbol Instance | The layer the override applies to. It will be an immutable version of the layer. |
editableboolean | If the value of the override can be changed. |
selectedboolean / undefined | If the override is selected (or undefined if it’s the override of a Symbol Source). |
Get the frame of an Override
var frame = override.getFrame()
The frame of an override can be different than the frame of its affected Layer in case where the Symbol Instance has been scaled for example.
Returns
A Rectangle describing the frame of the affected layer in the Symbol Instance’s coordinates.
Flow
var Flow = require('sketch/dom').Flow
The prototyping action associated with a layer.
Properties | |
---|---|
targetArtboard / Flow.BackTarget | The target artboard of the action or Flow.BackTarget if the action is a back action |
targetIdstring / Flow.BackTarget | The ID of target artboard of the action or Flow.BackTarget if the action is a back action |
animationTypeAnimationType | The type of the animation. |
Create a new prototyping action
layer.flow = {
target: artboard,
}
You can create an action without specifying an animation type, it will use the default one.
layer.flow = {
targetId: artboard.id,
}
You can create an action by using the ID of an Artboard instead of the artboard.
layer.flow = {
target: artboard,
animationType: Flow.AnimationType.slideFromLeft,
}
You can also specify the animation type.
layer.flow = {
target: Flow.BackTarget,
}
You can also create a back action.
Check if the action is a Back action
layer.flow.isBackAction()
Returns whether the prototyping action is a back action or not, eg. whether layer.flow.target === Flow.BackTarget
.
Check if the target is valid
layer.flow.isValidConnection()
In some cases, the target of the action can be invalid, for example when the target has been removed from the document. The methods returns whether the target is valid or not.
Flow.BackTarget
layer.flow = {
target: Flow.BackTarget,
}
Flow.BackTarget
is a constant that you can set the target to in order to always take you back to the last Artboard you were looking at. When a Target has been set to Flow.BackTarget
, the transition leading into it will be reversed on return.
Flow.AnimationType
Flow.AnimationType.slideFromLeft
Enumeration of the animation types.
Value | |
---|---|
none |
No animation |
slideFromLeft |
Slide from the left |
slideFromRight |
Slide from the right |
slideFromBottom |
Slide from the bottom |
slideFromTop |
Slide from the top |
Export Format
An export format associated with a layer.
Properties | |
---|---|
fileFormatstring | The file format of the export. |
prefixstring / undefined |
The prefix added to the file name. |
suffixstring / undefined |
The suffix added to the file name. |
sizestring | The size of the export. Valid values include 2x , 100w , 100width , 100px , 300h , 300height . |
Valid export file formats
jpg
png
tiff
eps
pdf
webp
svg
Selection
var selection = document.selectedLayers
A utility class to represent the layers selection. Contains some methods to make interacting with a selection easier.
Properties | |
---|---|
layersLayer[] | The Layers in the selection. Setting this property will change the selection. |
lengthnumber - read-only | The number of Layers in the selection. |
isEmptyboolean - read-only | Does the selection contain any layers? |
map
, forEach
, and reduce
selection.forEach(layer => log(layer.id))
selection.map(layer => layer.id)
selection.reduce((initial, layer) => {
initial += layer.name
return initial
}, '')
Even though a selection isn’t an array, it defines map
, forEach
and reduce
by just forwarding the arguments to its layers. Those are just convenience methods to avoid getting the layers every time.
Clear the Selection
selection.clear()
Clears the selection.
Returns
Return the selection (useful if you want to chain the calls).
Point
A utility class to represent a point.
Properties | |
---|---|
xnumber / Point | The x coordinate of the point. |
ynumber | The y coordinate of the point. |
Get a CGPoint
var cgPoint = point.asCGPoint()
Return the Point as a CGPoint
.
Get an NSPoint
var nsPoint = rect.asNSPoint()
Return the Point as a NSPoint
.
CurvePoint
A utility class to represent a curve point (with handles to control the curve in a path).
Properties | |
---|---|
pointPoint | The position of the point. |
curveFromPoint | The position of the handle control point for the incoming path. |
curveToPoint | The position of the handle control point for the outgoing path. |
cornerRadiusnumber | The corder radius of the point. |
pointTypePointType | The type of the point. |
Check if the point is selected
shape.points[0].isSelected()
In case the user is currently editing a path, you can check if a curve point is selected using the curvePoint.isSelected()
method.
CurvePoint.PointType
Enumeration of the animation types.
Value |
---|
Undefined |
Straight |
Mirrored |
Asymmetric |
Disconnected |
Rectangle
var Rectangle = require('sketch/dom').Rectangle
var rect = new Rectangle(0, 0, 100, 100)
var rectFromAnotherRect = new Rectangle(rect)
A utility class to represent a rectangle. Contains some methods to make interacting with a rectangle easier.
Properties | |
---|---|
xnumber / Rectangle | The x coordinate of the top-left corner of the rectangle. Or an object with {x, y, width, height} |
ynumber | The y coordinate of the top-left corner of the rectangle. |
widthnumber | The width of the rectangle. |
heightnumber | The height of the rectangle. |
Offset the Rectangle
var newRect = rect.offset(x, y)
Adjust the rectangle by offsetting it.
Returns
Return this rectangle (useful if you want to chain the calls).
Scale the Rectangle
var newRect = rect.scale(scaleWidth, scaleHeight)
Adjust the rectangle by scaling it. The scaleHeight
argument can be omitted to apply the same factor on both the width and the height.
Returns
Return this rectangle (useful if you want to chain the calls).
Change the coordinates basis
var newRect = rect.changeBasis({
from: layerA,
to: layerB,
})
var parentRect = rect.changeBasis({
from: layerA,
to: layerA.parent,
})
var pageRect = rect.changeBasis({
from: layerA,
// leaving out `to` means changing the
// basis to the Page's basis
})
Each layer defines its own system of coordinates (with its origin at the top left of the layer). You can change that basis from one layer to the other with changeBasis
.
Parameters | |
---|---|
changeobject - required | |
change.fromLayer | The layer in which the rectangle’s coordinates are expressed. |
change.toLayer | The layer in which the rectangle’s coordinates will be expressed. |
Both from
and to
can be omitted (but not at the same time) to change the basis from/to the Page coordinates.
Get a CGRect
var cgRect = rect.asCGRect()
Return the Rectangle as a CGRect
.
Get an NSRect
var nsRect = rect.asNSRect()
Return the Rectangle as a NSRect
.
fromNative
var sketch = require('sketch/dom')
var document = sketch.fromNative(context.document)
A utility function to get a wrapped object from a native Sketch model object.
Parameters | |
---|---|
objectNative Sketch Object | The native Sketch model object to wrap. |
Returns
The wrapped object of the right type (you can check is type with wrappedObject.type
), eg. a native document will be wrapped as a Document while a native text layer will be wrapped as a Text.
Assets
Wrapper classes that are used to represent reusable assets retrieved from a document or globally.
Color Asset
Properties | |
---|---|
namestring | The name of the asset, or null. |
colorstring | The hex string for the color. |
Get the Global Colors
var sketch = require('sketch/dom')
var colors = sketch.globalAssets.colors
Returns
An array of ColorAsset objects.
Gradient Asset
Properties | |
---|---|
namestring | The name of the asset, or null. |
gradientGradient | The gradient object. |
Get the Global Gradients
var sketch = require('sketch/dom')
var gradients = sketch.globalAssets.gradients
Returns
An array of GradientAsset objects.
Swatch
Properties | |
---|---|
namestring | The name of the swatch, or null. |
colorstring | The hex string for the color. |
Get a referencing Color
var sketch = require('sketch')
var mySwatch = sketch.Swatch.from({
name: 'Safety Orange',
color: '#ff6600'
})
var myColor = mySwatch.referencingColor
Returns
A Color that references a Color Variable, which you can use anywhere the API expects a Color object.
SmartLayout
The SmartLayout object contains the set of possible Smart Layouts that can be applied to SymbolMaster and Group layers.
Properties | |
---|---|
LeftToRight | Smart Layout flowing left to right |
HorizontallyCenter | Smart Layout expanding horizontally from the center |
RightToLeft | Smart Layout flowing right to left |
TopToBottom | Smart Layout flowing from top to bottom |
VerticallyCenter | Smart Layout expanding vertically from the center |
BottomToTop | Smart Layout flowing from bottom to top |
Set a Smart Layout
const SmartLayout = require('sketch').SmartLayout
layer.smartLayout = SmartLayout.TopToBottom
Given a reference to a SymbolMaster or Group layer use the smartLayout
setter to apply one of the Smart Layout values.
Clear a Smart Layout
Set the smartLayout
value to null
to remove the Smart Layout. This is the equivalent of selecting “None” in the Sketch Inspector.
symbol.smartLayout = null
Trigger a smart layout
const SmartLayout = require('sketch').SmartLayout
symbol.smartLayout = SmartLayout.TopToBottom
symbolInstance.resizeWithSmartLayout()
In order to trigger a Smart Layout resize in an instance, for example after changing an override value, call the resizeWithSmartLayout()
method on the SymbolInstance layer.
Layer
A Sketch layer. This is the base class for most of the Sketch components and defines methods to manipulate them.
Properties | |
---|---|
idstring | The unique ID of the Layer. |
namestring | The name of the Layer |
parentGroup | The group the layer is in. |
lockedboolean | If the layer is locked. |
hiddenboolean | If the layer is hidden. |
frameRectangle | The frame of the Layer. This is given in coordinates that are local to the parent of the layer. |
selectedboolean | If the layer is selected. |
flowFlow | The prototyping action associated with the layer. |
exportFormatsExportFormat[] | The export formats of the Layer. |
transformobject | The transformation applied to the Layer. |
transform.rotationnumber | The rotation of the Layer in degrees, clock-wise. |
transform.flippedHorizontallyboolean | If the layer is horizontally flipped. |
transform.flippedVerticallyboolean | If the layer is vertically flipped. |
Duplicate the Layer
var duplicatedLayer = layer.duplicate()
A new identical layer will be inserted into the parent of this layer.
Returns
A new Layer.
Remove the Layer
layer.remove()
Remove this layer from its parent.
Returns
The current layer (useful if you want to chain the calls).
Get the position in the hierarchy
var index = layer.index
The index of this layer in its parent. The layer at the back of the parent (visually) will be layer 0
. The layer at the front will be layer n - 1
(if there are n
layers).
Move the Layer in the hierarchy
Using the index
layer.index = 2
You can set the index of the layer to move it in the hierarchy.
Move to the front
layer.moveToFront()
// which is the same as
layer.index = layer.parent.layers.length - 1
Move this layer to the front of its parent.
Returns
The current layer (useful if you want to chain the calls).
Move forward
layer.moveForward()
// which is the same as
layer.index = layer.index + 1
Move this layer forward in its parent.
Returns
The current layer (useful if you want to chain the calls).
Move to the back
layer.moveToBack()
// which is the same as
layer.index = 0
Move this layer to the back of its parent.
Returns
The current layer (useful if you want to chain the calls).
Move backward
layer.moveBackward()
// which is the same as
layer.index = layer.index - 1
Move this layer backward in its parent.
Returns
The current layer (useful if you want to chain the calls).
Accessing the layer’s hierarchy
// access the page the layer is in
layer.getParentPage()
page.getParentPage() === undefined
// access the artboard the layer is in (if any)
layer.getParentArtboard()
artboard.getParentArtboard() === undefined
// access the Symbol Source the layer is in (if any)
layer.getParentSymbolMaster()
symbolMaster.getParentSymbolMaster() === undefined
// access the shape the layer is in (if any)
layer.getParentShape()
In addition to the direct parent
, you can access a few other entities in the hierarchy of the layer.
Group
var Group = require('sketch/dom').Group
A group of layers. It is also an instance of Layer so all the methods defined there are available.
Properties | |
---|---|
idstring | The unique ID of the Group. |
namestring | The name of the Group |
parentGroup | The group the Group is in. |
lockedboolean | If the Group is locked. |
hiddenboolean | If the Group is hidden. |
frameRectangle | The frame of the Group. This is given in coordinates that are local to the parent of the Layer. |
selectedboolean | If the Group is selected. |
flowFlow | The prototyping action associated with the Group. |
exportFormatsExportFormat[] | The export formats of the Group. |
transformobject | The transformation applied to the Group. |
transform.rotationnumber | The rotation of the Group in degrees, clock-wise. |
transform.flippedHorizontallyboolean | If the Group is horizontally flipped. |
transform.flippedVerticallyboolean | If the Group is vertically flipped. |
styleStyle | The style of the Group. |
sharedStyleSharedStyle / null |
The associated shared style or null . |
sharedStyleIdstring / null |
The ID of the SharedStyle or null , identical to sharedStyle.id . |
layersLayer[] | The Layers that this component groups together. |
smartLayoutSmartLayout | The Group’s Smart Layout. |
Create a new Group
new Group()
var group = new Group({
name: 'my name',
layers: [
{
type: sketch.Types.Text,
text: 'Hello world',
},
],
})
Adjust to fit its children
group.adjustToFit()
Adjust the group to fit its children.
Returns
The current group (useful if you want to chain the calls).
Page
var Page = require('sketch/dom').Page
A Sketch page. It is an instance of both Layer and Group so all the methods defined there are available.
Properties | |
---|---|
idstring | The unique ID of the Page. |
namestring | The name of the Page |
parentDocument | The document the page is in. |
layersLayer[] | An array of the layers and Artboards that this page has. It contains the first level children of the Page. If you need to access all layers and sublayers in the Page, use find . |
frameRectangle | The frame of the page. |
selectedboolean | If the Page is selected. |
Create a new Page
new Page()
new Page({
name: 'my page',
})
Get the selected Layers of the Page
var selection = document.selectedLayers
A read-only property to get the layers that the user has selected in the page.
Returns
Return a Selection object.
Symbols Page
The “Symbols” page is similar to other pages. The only way it is specific is when creating a Symbol, Sketch will ask the user if they want to move it to that page.
You can put Symbols in any page but if you want to respect the convention Sketch put in place, here are a few methods to help you do so.
Get the Symbols Page
var symbolsPage = Page.getSymbolsPage(document)
A method to get the Symbols Page of a Document.
Parameters | |
---|---|
documentDocument - required | The document from which you want the Symbols Page. |
Returns
Return a Page or undefined
if there is no Symbols Page yet.
Create the Symbols Page
var symbolsPage = Page.createSymbolsPage()
symbolsPage.parent = document
A method to create the Page with the name that Sketch will recognize as the Symbols Page.
Returns
Return a Page.
Knows if a Page is the Symbols Page
var isSymbolsPage = page.isSymbolsPage()
A method to tell if the page is the Symbols Page.
Returns
Return a boolean
.
Artboard
var Artboard = require('sketch/dom').Artboard
A Sketch artboard. It is an instance of both Layer and Group so all the methods defined there are available.
Properties | |
---|---|
idstring | The unique ID of the Artboard. |
namestring | The name of the Artboard |
parentPage | The page the Artboard is in. |
selectedboolean | If the Artboard is selected. |
layersLayer[] | The layers that this component groups together. |
frameRectangle | The frame of the Artboard. This is given in coordinates that are local to the parent of the layer. |
flowStartPointboolean | A Start Point allows you to choose where to start your prototype from. |
exportFormatsExportFormat[] | The export formats of the Artboard. |
backgroundobject | The background of the Artboard |
background.enabledboolean | If the background should be enabled, eg. shown or not |
background.includedInExportboolean | If the background should be exported or if it should be transparent during the export |
background.colorstring | The rgba representation of the color of the background |
Create a new Artboard
new Artboard()
new Artboard({
name: 'my name',
flowStartPoint: true,
})
Shape
var Shape = require('sketch/dom').Shape
A shape layer. It is an instance of Layer so all the methods defined there are available. It is shaped by its layers which have boolean operations between them.
Properties | |
---|---|
idstring | The unique ID of the Shape. |
namestring | The name of the Shape |
parentGroup | The group the shape is in. |
lockedboolean | If the Shape is locked. |
hiddenboolean | If the shape is hidden. |
frameRectangle | The frame of the Shape. This is given in coordinates that are local to the parent of the layer. |
selectedboolean | If the Shape is selected. |
flowFlow | The prototyping action associated with the Shape. |
exportFormatsExportFormat[] | The export formats of the Shape. |
transformobject | The transformation applied to the Layer. |
transform.rotationnumber | The rotation of the Layer in degrees, clock-wise. |
transform.flippedHorizontallyboolean | If the layer is horizontally flipped. |
transform.flippedVerticallyboolean | If the layer is vertically flipped. |
styleStyle | The style of the Shape. |
sharedStyleSharedStyle / null |
The associated shared style or null . |
sharedStyleIdstring / null |
The ID of the SharedStyle or null , identical to sharedStyle.id . |
Create a new Shape
new Shape({
name: 'my shape',
})
Image
var Image = require('sketch/dom').Image
An image layer. It is an instance of Layer so all the methods defined there are available.
Properties | |
---|---|
idstring | The unique ID of the Image. |
namestring | The name of the Image |
parentGroup | The group the Image is in. |
lockedboolean | If the Image is locked. |
hiddenboolean | If the Image is hidden. |
frameRectangle | The frame of the Image. This is given in coordinates that are local to the parent of the layer. |
selectedboolean | If the Image is selected. |
flowFlow | The prototyping action associated with the Image. |
exportFormatsExportFormat[] | The export formats of the Image. |
transformobject | The transformation applied to the Image. |
transform.rotationnumber | The rotation of the Image in degrees, clock-wise. |
transform.flippedHorizontallyboolean | If the Image is horizontally flipped. |
transform.flippedVerticallyboolean | If the Image is vertically flipped. |
styleStyle | The style of the Image. |
sharedStyleSharedStyle / null |
The associated shared style or null . |
sharedStyleIdstring / null |
The ID of the SharedStyle or null , identical to sharedStyle.id . |
imageImageData | The actual image of the layer. |
Create a new Image
var imageLayer = new Image({
image: 'path/to/image.png',
})
The image property accepts a wide range of input:
- an
ImageData
- a
Buffer
- a native
NSImage
- a native
NSURL
- a native
MSImageData
- a string: path to the file to load the image from
- an object with a
path
property: path to the file to load the image from - an object with a
base64
string: a base64 encoded image
var imageLayer = new Image({
image: 'path/to/image.png',
frame: new Rectangle(0, 0, 300, 200),
})
By default, an Image
layer will be created with a size of 100 × 100 pixels, unless you provide a frame
property on its constructor.
Original Size
imageLayer.resizeToOriginalSize()
Adjust the Image to its original size. This is equivalent to pressing the ‘Original Size’ button in Sketch’s Inspector.
Returns
The current Image (useful if you want to chain the calls).
For performance reasons, Sketch initializes the Image
object lazily. So if you want to set the dimensions of your Image layer to those of the original file, you’ll first need to create the object, and then call the resizeToOriginalSize
method.
ImageData
var imageData = imageLayer.image
imageData.nsimage // return a native NSImage
imageData.nsdata // return a native NSData representation of the image
An ImageData
is a wrapper around a native NSImage
.
You can access the native NSImage
with nsimage
or a native NSData
representation of the image with nsdata
.
imageLayer.image.size // { width: 100, height: 100 }
As a convenience, you can access the original size of an ImageData
object via its size
property.
ShapePath
var ShapePath = require('sketch/dom').ShapePath
A shape path layer. It is an instance of Layer so all the methods defined there are available.
Properties | |
---|---|
idstring | The unique ID of the ShapePath. |
namestring | The name of the ShapePath |
parentGroup | The group the ShapePath is in. |
lockedboolean | If the ShapePath is locked. |
hiddenboolean | If the ShapePath is hidden. |
frameRectangle | The frame of the ShapePath. This is given in coordinates that are local to the parent of the layer. |
selectedboolean | If the ShapePath is selected. |
flowFlow | The prototyping action associated with the ShapePath. |
exportFormatsExportFormat[] | The export formats of the ShapePath. |
styleStyle | The style of the ShapePath. |
sharedStyleSharedStyle / null |
The associated shared style or null . |
sharedStyleIdstring / null |
The ID of the SharedStyle or null , identical to sharedStyle.id . |
shapeTypeShapeType | The type of the Shape Path. It can only be set when creating a new ShapePath. |
pointsCurvePoint[] | The points defining the Shape Path. |
closedboolean | If the Path is closed. |
Create a new ShapePath
const shapePath = new ShapePath({
name: 'my shape path',
shapeType: ShapePath.ShapeType.Oval,
})
You can only set the shapeType
when creating a new one. Once it is created, the shapeType
is read-only. If it is not specified and you do not specify any points
, it will default to ShapePath.ShapeType.Rectangle
(if you do specify some points
, it will default to ShapePath.ShapeType.Custom
).
const shapePath = ShapePath.fromSVGPath('M10 10 H 90 V 90 H 10 L 10 10')
You can also create a new ShapePath from an SVG path (the string that goes in the d
attribute of a path
tag in an SVG). See the MDN documentation for more information about SVG paths.
Get the SVG path
const svgPath = shapePath.getSVGPath()
Returns a string representing the SVG path of the ShapePath.
ShapePath.ShapeType
ShapePath.ShapeType.Oval
Enumeration of the type of Shared Style.
Value |
---|
Rectangle |
Oval |
Triangle |
Polygon |
Star |
Custom |
ShapePath.PointType
ShapePath.PointType.Undefined
Enumeration of the point types.
Value |
---|
Undefined |
Straight |
Mirrored |
Asymmetric |
Disconnected |
Text
var Text = require('sketch/dom').Text
A text layer. It is an instance of Layer so all the methods defined there are available.
Properties | |
---|---|
idstring | The unique ID of the Text. |
namestring | The name of the Text |
parentGroup | The group the Text is in. |
lockedboolean | If the Text is locked. |
hiddenboolean | If the Text is hidden. |
frameRectangle | The frame of the Text. This is given in coordinates that are local to the parent of the layer. |
selectedboolean | If the Text is selected. |
flowFlow | The prototyping action associated with the Text. |
exportFormatsExportFormat[] | The export formats of the Symbol Source. |
transformobject | The transformation applied to the Text. |
transform.rotationnumber | The rotation of the Text in degrees, clock-wise. |
transform.flippedHorizontallyboolean | If the Text is horizontally flipped. |
transform.flippedVerticallyboolean | If the Text is vertically flipped. |
styleStyle | The style of the Text. |
sharedStyleSharedStyle / null |
The associated shared style or null . |
sharedStyleIdstring / null |
The ID of the SharedStyle or null , identical to sharedStyle.id . |
textstring | The string value of the text layer. |
lineSpacingLineSpacing | The line spacing of the layer. |
fixedWidthboolean | Whether the layer should have a fixed width or a flexible width. |
Create a new Text
var text = new Text({
text: 'my text',
alignment: Text.Alignment.center,
})
Adjust to fit its value
text.adjustToFit()
Adjust the Text to fit its value.
Returns
The current Text (useful if you want to chain the calls).
fragments
var fragments = text.fragments
Returns a array of the text fragments for the text. Each one is a object containing a rectangle, a baseline offset, the range of the fragment, and the substring {rect, baselineOffset, range, text}
.
Text.Alignment
Text.Alignment.center
Enumeration of the alignments of the text.
Value | |
---|---|
left |
Visually left aligned |
right |
Visually right aligned |
center |
Visually centered |
justify |
Fully-justified. The last line in a paragraph is natural-aligned. |
Text.VerticalAlignment
Text.VerticalAlignment.center
Enumeration of the vertical alignments of the text.
Value | |
---|---|
top |
Visually top aligned |
center |
Visually vertically centered |
bottom |
Visually bottom aligned |
Text.LineSpacing
Text.LineSpacing.constantBaseline
Enumeration of the line spacing behavior for the text.
Value | |
---|---|
constantBaseline |
Uses min & max line height on paragraph style |
variable |
Uses MSConstantBaselineTypesetter for fixed line height |
Symbol Source
var SymbolMaster = require('sketch/dom').SymbolMaster
A Symbol Source. It is an instance of Artboard (hence of Layer and Group) so all the methods defined there are available.
Properties | |
---|---|
idstring | The unique ID of the Symbol Source object (not to be confused with symbolId ). |
namestring | The name of the Symbol Source |
parentGroup | The group the Symbol Source is in. |
frameRectangle | The frame of the Symbol Source. This is given in coordinates that are local to the parent of the layer. |
selectedboolean | If the Symbol Source is selected. |
exportFormatsExportFormat[] | The export formats of the Symbol Source. |
layersLayer[] | The layers composing the Symbol Source. |
backgroundobject | The background of the Symbol Source |
background.enabledboolean | If the background should be enabled, eg. shown or not |
background.includedInExportboolean | If the background should be exported or if it should be transparent during the export |
background.includedInInstanceboolean | If the background should appear in the instances of the Symbol Source |
background.colorstring | The rgba representation of the color of the background |
symbolIdstring | The unique ID of the Symbol that the Source and its instances share. |
overridesOverride[] | The array of the overrides that the instances of the Symbol Source will be able to change. |
Create a new Symbol Source
var symbol = new SymbolMaster({
name: 'my symbol',
})
Create a new Symbol Source from an Artboard
var symbol = SymbolMaster.fromArtboard(artboard)
Replace the artboard with a Symbol Source.
Parameters | |
---|---|
artboardArtboard - required | The artboard to create the Symbol Source from. |
Returns
A new SymbolMaster
Change to an Artboard
var artboard = symbol.toArtboard()
Replace the Symbol Source with an artboard and detach all its instances converting them into groups.
Returns
A new Artboard
Create a new Instance
var instance = symbol.createNewInstance()
Creates a new SymbolInstance linked to this Source, ready for inserting in the document.
Returns
A new SymbolInstance
Get all the Instances
var instances = symbol.getAllInstances()
Returns an array of all instances of the Symbol in the document, on all pages.
Get Library defining the Symbol Source
var originLibrary = symbol.getLibrary()
If the Symbol Source was imported from a library, the method can be used to:
- know about it
- get the library back
Returns
The Library the Symbol was defined in, or null
if it is a local symbol.
Sync the local reference with the library version
const success = symbol.syncWithLibrary()
If a Library has some updates, you can synchronize the local Symbol Source with the Library’s version and bypass the panel where the user chooses the updates to bring.
Returns
true
if it succeeded.
Unlink the local reference from the library
const success = symbol.unlinkFromLibrary()
You can unlink a Symbol Source from the Library it comes from and make it a local Symbol Source instead. It will be added to the Symbols
Page.
Returns
true
if it succeeded.
Symbol Instance
var SymbolInstance = require('sketch/dom').SymbolInstance
A Symbol instance. It is an instance of Layer so all the methods defined there are available.
Properties | |
---|---|
idstring | The unique ID of the Symbol Instance object (not to be confused with symbolId ). |
namestring | The name of the Symbol Instance |
parentGroup | The group the Symbol Instance is in. |
lockedboolean | If the Symbol Instance is locked. |
hiddenboolean | If the Symbol Instance is hidden. |
frameRectangle | The frame of the Symbol Instance. This is given in coordinates that are local to the parent of the layer. |
flowFlow | The prototyping action associated with the Symbol. |
selectedboolean | If the Symbol Instance is selected. |
exportFormatsExportFormat[] | The export formats of the Symbol Instance. |
transformobject | The transformation applied to the Symbol Instance. |
transform.rotationnumber | The rotation of the Symbol Instance in degrees, clock-wise. |
transform.flippedHorizontallyboolean | If the Symbol Instance is horizontally flipped. |
transform.flippedVerticallyboolean | If the Symbol Instance is vertically flipped. |
styleStyle | The style of the Symbol Instance. |
symbolIdstring | The unique ID of the Symbol that the instance and its master share. |
masterSymbolMaster | The Symbol Source that the instance is linked to. |
overridesOverride[] | The array of the overrides to modify the instance. |
Create a new Symbol Instance
var instance = new SymbolInstance({
name: 'my symbol instance',
symbolId: symbolId,
})
Create a new Symbol Instance from a Symbol Source
var instance = symbol.createNewInstance()
Creates a new SymbolInstance linked to this Source, ready for inserting in the document.
Returns
A new SymbolInstance
Detach the instance
var group = instance.detach()
var group = instance.detach({
recursively: true,
})
Replaces a group that contains a copy of the Symbol this instance refers to. Returns null
if the Source contains no layers instead of inserting an empty group
Parameters | |
---|---|
optionsobject | The options to apply when detaching the instance. |
options.recursivelyboolean | If it should detach the nested symbols as well. Default to false . |
Returns
A new Group or null
Set an Override value
instance.setOverrideValue(instance.overrides[0], 'overridden')
Change the value of the override.
Parameters | |
---|---|
overrideOverride - required | The override to change. |
valuestring / NSImage - required | The value of override to set. Can be a string or an NSImage or a symbolId depending on the type of the override. |
Returns
The current Symbol instance (useful if you want to chain the calls).
Resize with Smart Layout
instance.resizeWithSmartLayout()
In order to trigger a Smart Layout resize in an instance, for example after changing an override value, call the resizeWithSmartLayout()
method.
HotSpot
var HotSpot = require('sketch/dom').HotSpot
A Sketch hotspot. It is an instance of Layer so all the methods defined there are available.
Properties | |
---|---|
idstring | The unique ID of the HotSpot. |
namestring | The name of the HotSpot |
parentGroup | The group the HotSpot is in.. |
lockedboolean | If the HotSpot is locked. |
hiddenboolean | If the HotSpot is hidden. |
frameRectangle | The frame of the HotSpot. This is given in coordinates that are local to the parent of the HotSpot. |
selectedboolean | If the HotSpot is selected. |
flowFlow | The prototyping action associated with the HotSpot. |
Create a new Hotspot
new HotSpot()
new HotSpot({
name: 'my name',
flow: {
target: artboard,
},
})
Create a new Hotspot from a Layer
var hotspot = HotSpot.fromLayer(layer)
Slice
var Slice = require('sketch/dom').Slice
A Sketch slice. It is an instance of Layer so all the methods defined there are available.
Properties | |
---|---|
idstring | The unique ID of the Slice. |
namestring | The name of the Slice |
parentGroup | The group the Slice is in. |
lockedboolean | If the Slice is locked. |
hiddenboolean | If the Slice is hidden. |
frameRectangle | The frame of the Slice. This is given in coordinates that are local to the parent of the layer. |
selectedboolean | If the Slice is selected. |
exportFormatsExportFormat[] | The export formats of the Slice. |
Settings
var Settings = require('sketch/settings')
A set of functions to handle user settings. The settings are persisted when the user closes Sketch.
Get a plugin setting
var setting = Settings.settingForKey('my-key')
Return the value of a setting scoped to your plugin for a given key.
Parameters | |
---|---|
keystring - required | The setting to look up. |
Returns
The setting that was stored for the given key. undefined
if there was nothing.
Set a plugin setting
Settings.setSettingForKey('my-key', 0.1)
Store a value of a setting scoped to your plugin for a given key.
Parameters | |
---|---|
keystring - required | The setting to set. |
valueany - required | The value to set it to. |
Get a Sketch setting
var setting = Settings.globalSettingForKey('my-key')
Return the value of a Sketch setting for a given key.
Parameters | |
---|---|
keystring - required | The setting to look up. |
Returns
The setting that was stored for the given key. undefined
if there was nothing.
Set a Sketch setting
Settings.setGlobalSettingForKey('my-key', 0.1)
Store a value of a Sketch setting for a given key.
Parameters | |
---|---|
keystring - required | The setting to set. |
valueany - required | The value to set it to. |
Get a Layer setting
var setting = Settings.layerSettingForKey(layer, 'my-key')
Return the value of a setting for a given key on a specific Layer or DataOverride or Override.
Parameters | |
---|---|
layerLayer / DataOverride / Override - required | The layer on which a setting is stored. |
keystring - required | The setting to look up. |
Returns
The setting that was stored for the given key. undefined
if there was nothing.
Set a Layer setting
Settings.setLayerSettingForKey(layer, 'my-key', 0.1)
Store a value of a setting for a given key on a specific Layer or DataOverride or Override.
Parameters | |
---|---|
layerLayer / DataOverride / Override - required | The layer on which the setting is set. |
keystring - required | The setting to set. |
valueany - required | The value to set it to. |
Get a Document setting
var setting = Settings.documentSettingForKey(document, 'my-key')
Return the value of a setting for a given key on a specific document.
Parameters | Description |
---|---|
documentDocument - required | The document on which a setting is stored. |
keystring - required | The setting to look up. |
Returns
The setting that was stored for the given key. undefined
if there was nothing.
Set a Document setting
Settings.setDocumentSettingForKey(document, 'my-key', 0.1)
Store a value of a setting for a given key on a specific document.
Parameters | |
---|---|
documentDocument - required | The document on which the setting is set. |
keystring - required | The setting to set. |
valueany - required | The value to set it to. |
Get a session variable
var myVar = Settings.sessionVariable('myVar')
Return the value of a variable which is persisted when the plugin finishes to run but is not persisted when Sketch closes. It is useful when you want to keep a value between plugin’s runs.
Parameters | |
---|---|
keystring - required | The variable to look up. |
Returns
The setting that was stored for the given key. undefined
if there was nothing.
Set a session variable
Settings.setSessionVariable('myVar', 0.1)
Store a value of a variable which is persisted when the plugin finishes to run but is not persisted when Sketch closes. It is useful when you want to keep a value between plugin’s runs.
Parameters | |
---|---|
keystring - required | The variable to set. |
valueany - required | The value to set it to. |
UI
var UI = require('sketch/ui')
A set of functions to show some user interfaces. The set is small on purpose. Any more complex UI should be provided by third party libraries and doesn’t need to be in the core.
Show a message
UI.message('Hello world!')
Show a small, temporary, message to the user. The message appears at the bottom of the selected document, and is visible for a short period of time. It should consist of a single line of text.
Parameters | |
---|---|
textstring - required | The message to show. |
documentDocument | The document to show the message into. |
Show an alert
UI.alert('my title', 'Hello World!')
Show an alert with a custom title and message. The alert is modal, so it will stay around until the user dismisses it by pressing the OK button.
Parameters | |
---|---|
titlestring - required | The title of the alert. |
textstring - required | The text of the message. |
Get an input from the user
UI.getInputFromUser(
"What's your name?",
{
initialValue: 'Appleseed',
},
(err, value) => {
if (err) {
// most likely the user canceled the input
return
}
}
)
UI.getInputFromUser(
"What's your favorite design tool?",
{
type: UI.INPUT_TYPE.selection,
possibleValues: ['Sketch', 'Paper'],
},
(err, value) => {
if (err) {
// most likely the user canceled the input
return
}
}
)
Shows a simple input sheet which displays a message, and asks for an input from the user.
Parameters | |
---|---|
messagestring - required | The prompt message to show. |
optionsobject | Options to customize the input sheet. Most of the options depends on the type of the input. |
option.descriptionstring | A secondary text to describe with more details the input. |
option.typeInput Type | The type of the input. |
option.initialValuestring / number | The initial value of the input. |
option.possibleValuesstring[] - required with a selection | The possible choices that the user can make. Only used for a selection input. |
option.numberOfLinesnumber | Controls the height of the input field. Only used for a string input. If a value is provided it converts the textfield into a scrollable textarea. |
callbackfunction | A function called after the user entered the input. It is called with an Error if the user canceled the input and a string or number depending on the input type (or undefined ). |
UI.INPUT_TYPE
UI.INPUT_TYPE.selection
The enumeration of the different input types.
Values |
---|
string |
selection |
Get the theme of Sketch
var theme = UI.getTheme()
if (theme === 'dark') {
// shows UI in dark theme
} else {
// shows UI in light theme
}
Sketch has 2 themes: light
and dark
. If your plugin has some custom UI, it should support both as well.
Data Supplier
var DataSupplier = require('sketch/data-supplier')
When your plugin supplies some data, don’t forget to set the suppliesData
field to true
in your manifest.json
!
Register a Data Supplier
var DataSupplier = require('sketch/data-supplier')
// onStartup would be the handler for the `Startup` action defined in the manifest.json
export function onStartup() {
DataSupplier.registerDataSupplier(
'public.text',
'My Custom Data',
'SupplyKey'
)
}
Register some data with a name and a key.
Parameters | |
---|---|
dataTypestring - required | The data type. Allowed values are public.text , public.image , or public.json (available in Sketch 71+). |
dataNamestring - required | The data name, used as the menu item title for the data. |
actionstring - required | The name of the Action that will be dispatched when the user requests some data. See supplyData . |
Context of the action
When a user runs a Data plugin, Sketch will forward the request to your plugin, passing a context.data
object with all the information you need to fulfil the request:
Key | |
---|---|
context.data.key |
A unique key to identify the supply request. You need to pass it to the supply method untouched. |
context.data.items |
The array of native model objects for which we want some data. It can be either a native Text, a native Shape or a native DataOverride (a special object when the data is for an Override) |
var util = require('util')
var sketch = require('sketch/dom')
var DataSupplier = require('sketch/data-supplier')
// onSupplyKeyNeeded would be the handler for
// the `SupplyKey` action defined in the manifest.json
export function onSupplyKeyNeeded(context) {
var count = context.data.items.count()
var key = context.data.key
var items = context.data.items
// you will often want to get wrapped objects instead
// of the native ones supplied in the context
var wrappedItems = util.toArray(items).map(sketch.fromNative)
}
Supply the Data
You have two different methods to supply data from your plugin: supplyData
(to provide all the data at once), and supplyDataAtIndex
(to provide data one by one).
Supply all the data in one go
var DataSupplier = require('sketch/data-supplier')
// onSupplyKeyNeeded would be the handler for
// the `SupplyKey` action defined in the manifest.json
export function onSupplyKeyNeeded(context) {
var count = context.data.items.count()
var key = context.data.key
var data = Array.from(Array(count)).map(i => 'foo')
DataSupplier.supplyData(key, data)
}
When the plugin providing the dynamic data has finished generating the data (could be an asynchronous operation), it will call this function with the data key and the data.
Parameters | |
---|---|
keystring - required | Should be equal to context.data.key |
datastring[] - required | The list of values to provide. In case of public.image , the string is the path to the image. |
Supply the data one by one
var util = require('util')
var sketch = require('sketch/dom')
var DataSupplier = require('sketch/data-supplier')
// onSupplyKeyNeeded would be the handler for
// the `SupplyKey` action defined in the manifest.json
export function onSupplyKeyNeeded(context) {
var key = context.data.key
var items = util.toArray(context.data.items).map(sketch.fromNative)
items.forEach((item, i) => {
// item is either a Layer or a DataOverride
DataSupplier.supplyDataAtIndex(key, 'foo', i)
})
}
When the plugin providing the dynamic data has finished generating the datum (could be an asynchronous operation), it will call this function with the data key and the datum.
Parameters | |
---|---|
keystring - required | Should be equal to context.data.key |
datumstring - required | The value to provide. In case of public.image , the string is the path to the image. In case of public.json , the value is a JSON object. |
indexnumber - required | The index of the item you are providing a value for. |
DataOverride
A special object passed in the context of the action to supply data when the item is an Override.
Properties | |
---|---|
idstring | The name of the override. |
overrideOverride | The override whose value will replaced by the supplied data. |
symbolInstanceSymbolInstance | The Symbol instance that the override is on that will have the data replaced. |
Deregister your Data Suppliers
var DataSupplier = require('sketch/data-supplier')
// onShutdown would be the handler for the `Shutdown` action defined in the manifest.json
export function onShutdown() {
DataSupplier.deregisterDataSuppliers()
}
When registering something, it’s good practice to clean up after it. This is specially useful when your plugin is updated: the Shutdown
Action will be called before the Startup
will. It gives you the opportunity to update your handler cleanly.
async
var fiber = require('sketch/async').createFiber()
longRunningTask(function(err, result) {
fiber.cleanup()
// you can continue working synchronously here
})
By default, Sketch cleans up your script as soon as its call-stack is empty. So if you schedule an asynchronous task, chances are that when the task returns, your script will be cleaned up and it will crash Sketch.
A fiber is a way to keep track of a asynchronous task. The script will stay alive as long as at least one fiber is running.
To end a fiber, call fiber.cleanup()
. This will tell Sketch that it can garbage collect the script if no other fiber is running.
You can run a function when the fiber is about to be cleaned up by setting a callback: fiber.onCleanup(function () {...})
. Always do your clean up in this function instead of doing before calling fiber.cleanup
: there might be some cases where the fiber will be cleaned up by Sketch so you need to account for that.
export
var sketch = require('sketch/dom')
sketch.export(layer, {
formats: 'svg',
})
sketch.export([layer, layer2])
const options = { scales: '1, 2, 3', formats: 'png, jpg' }
sketch.export(page, options)
sketch.export(document.pages)
const options = { formats: 'json', output: false }
const sketchJSON = sketch.export(layer, options)
const options = { formats: 'png', output: false }
const buffer = sketch.export(layer, options)
Export an object, using the options supplied.
Parameters | |
---|---|
objectToExportLayer / Layer[] / Page / Page[] | The object to export. |
optionsobject | Options indicating which sizes and formats to use, etc.. |
options.outputstring | this is the path of the folder where all exported files are placed (defaults to "~/Documents/Sketch Exports" ). If falsey, the data for the objects are returned immediately. |
options.formatsstring | Comma separated list of formats to export to (png , jpg , webp , tiff , svg , eps , pdf or json ) (default to "png" ). |
options.scalesstring | Comma separated list of scales which determine the sizes at which the layers are exported (defaults to "1" ). |
options[‘use-id-for-name’]boolean | Name exported images using their id rather than their name (defaults to false ). |
options[‘group-contents-only’]boolean | Export only layers that are contained within the group (default to false ). |
options.overwritingboolean | Overwrite existing files (if any) with newly generated ones (defaults to false ). |
options.trimmedboolean | Trim any transparent space around the exported image (leaving it undefined will match Sketch’s behavior: trim for layers that do not have a background color). |
options[‘save-for-web’]boolean | If exporting a PNG, remove metadata such as the colour profile from the exported file (defaults to false ). |
options.compactboolean | If exporting a SVG, make the output more compact (defaults to false ). |
options[‘include-namespaces’]boolean | If exporting a SVG, include extra attributes (defaults to false ). |
options.progressiveboolean | If exporting a JPG, export a progressive JPEG (only used when exporting to jpeg ) (defaults to false ). |
options.compressionnumber | If exporting a JPG, the compression level to use fo jpeg (with 0 being the completely compressed, 1.0 no compression) (defaults to 1.0 ). |
The method returns:
undefined
ifoptions.output
isundefined
or a string- an array of
Buffer
s ifobjectToExport
is an array andoptions.formats
is an image format - an array of
Object
s ifobjectToExport
is an array andoptions.formats
isjson
- a
Buffer
ifobjectToExport
is a single item andoptions.formats
is an image format - a
Object
ifobjectToExport
is a single item andoptions.formats
isjson
import
var sketch = require('sketch/dom')
const layer = sketch.createLayerFromData(buffer, 'svg')
const svgString =
'<svg width="200px" height="100px" viewBox="0 0 200 100" version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"><rect fill="#000000" x="0" y="0" width="200" height="100"></rect></svg>'
const group = sketch.createLayerFromData(svgString, 'svg')
Import a file as a Layer.
Parameters | |
---|---|
dataBuffer / string | The data for the file. |
typestring | The type of the file being imported. "svg" , "pdf" , "eps" , or "bitmap" . |
The method returns:
find
var sketch = require('sketch/dom')
sketch.find('Shape')
// find all the Shapes in the current Document
sketch.find('Shape')
// find all the Layers in the first Page of the Document
sketch.find('*', document.pages[0])
// find all the Layers named "Layer-Name"
sketch.find('[name="Layer-Name"]')
// find all the Shape named "Layer-Name"
sketch.find('Shape, [name="Layer-Name"]')
⚠️ This API is in preview. It might change in the future depending on the feedback from the community.
Find Layers fitting some criteria.
Parameters | |
---|---|
selectorstring - required | The object to export. |
scopeGroup / Document | The scope of the search. By default it is the current Document. |